
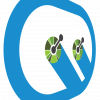
API Framework
Everything is an (Open)API in .NET
Framework on top of ASP.NET Core that aims to make building OpenAPI systems more flexible

More options for building APIs
POCOs, Nuget Packages, Roslyn scripts, Delegates... With API Framework you have more options than just the controllers for building APIs in ASP.NET Core.

24/7 Backends
Create ASP.NET Core backends which can be updated runtime. APIs and endpoints can be added and updated when the system is running.

Integration Layers
API Framework can be used as the secure OpenAPI gateway into other systems. With plugins you can generate OpenAPI endpoints from databases, file systems, Azure blob storage, and more.
Features
Here’s some of the main features of API Framework
Everything is an OpenAPI
The API can be anything: Database, local files. Even the web browser. APIs can be created with C#, Roslyn Scripts and using delegates.
Conventions & Configuration
Code based configuration or appsettings.json are both supported. Auto mode lets API Framework to create endpoints automatically.
Runtime Changes
APIs and endpoints can be configured runtime, when the application is running. No need to restart the system to add a new endpoint.
API Factories
API Framework's power comes from the API Factories. The API Factory allows the creation of an API runtime. This often includes code generation.
Health Checks
Each endpoint can be monitored using a health check.
Plugin Support
API Frameworks supports plugins. Nuget.org contains multiple ready made plugins, like SQL Server and Local Files. Custom plugins can be created and shared using Nuget.
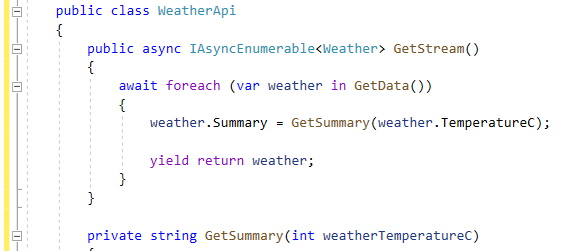
Async JSON Streams
Built-in support for returning IAsyncEnumerable from your APIs. This helps with the TTFB as the client receives the first bytes of the response quickly.
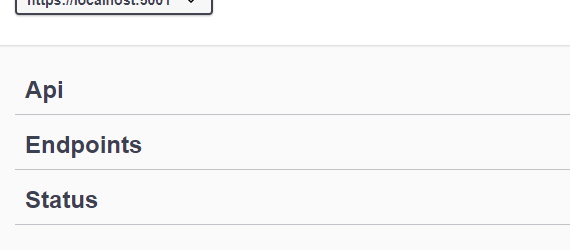
Built-in Admin Endpoints
API Framework comes with built-in Admin endpoints. These can be used to monitor the status of the system or to add and remove endpoints runtime.
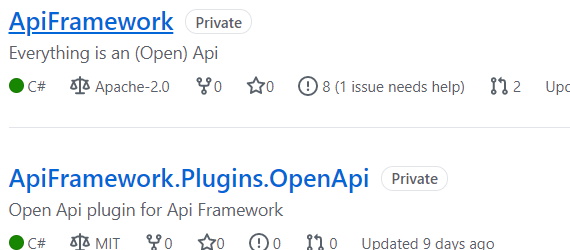
Open Source & Open Standards
API Framework is Apache 2.0 licensed and available from GitHub.
Getting Started: Few steps are required in order to use the framework.
Step 1: Installing Api Framework
Api Framework is available through Nuget:
Api Framework can be installed into an existing ASP.NET Core app. It works together with Controllers and Actions. If you create a new app, Web Api template is a good starting point. When you have the package installed, use ConfigureServices to add Api Framework into your app:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddApiFramework();
}
Step 2: Creating Apis
Next you create your APIs. Each Api has two properties: Name and Version.
Most often API is a C# class or a .NET type. But you can also use assemblies, Nuget packages, Roslyn scripts and delegates as APIs. There’s many APIs available as plugins through Nuget.
Here we create an API from a func and give it a name “MyApi”. The API defaults to version 1.0.0.0 if no version is specified.
services.AddApiFramework()
.AddApi(new Func<string>(() =>
"Hello from Our Custom Delegate Api"),
"MyApi");
Step 3: Creating Endpoints
And finally you create endpoints from your APIs. Endpoint gives your API a route (for example ‘/helloworld’) and a configuration (if needed).
You can create multiple endpoints from each API and every endpoint can have different configuration.
In our example we create two endpoints from our API: /hello and /another. Our “MyApi” doesn’t require a configuration:
services.AddApiFramework()
.AddApi(new Func<string>(() => "Hello from Our Custom Delegate Api"), "MyApi")
.AddEndpoint("/hello", "MyApi")
.AddEndpoint("/another", "MyApi");
Step 4: Running the App
Now when you run your app, the endpoints are available from /api/hello and /api/another.
Api Framework by default uses a base address /api and all the endpoints are prefixed with it.
This was just a quick summary. To start building powerful APIs, please visit the documentation to find more details.
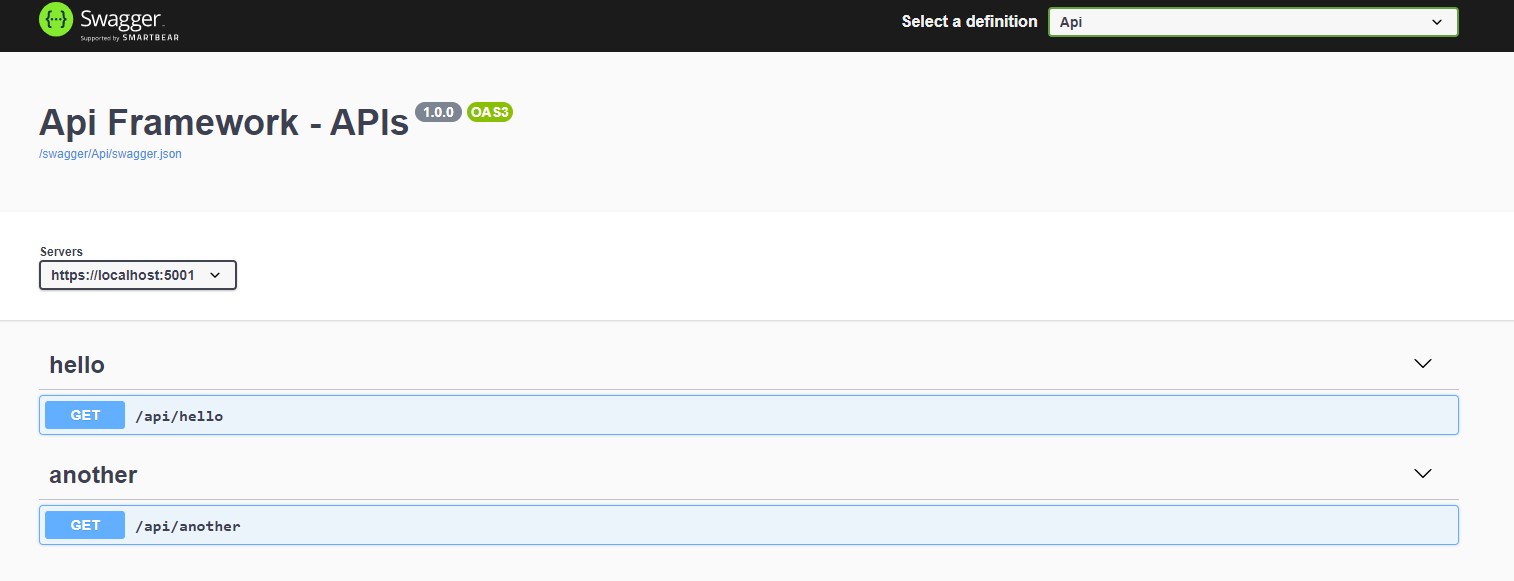
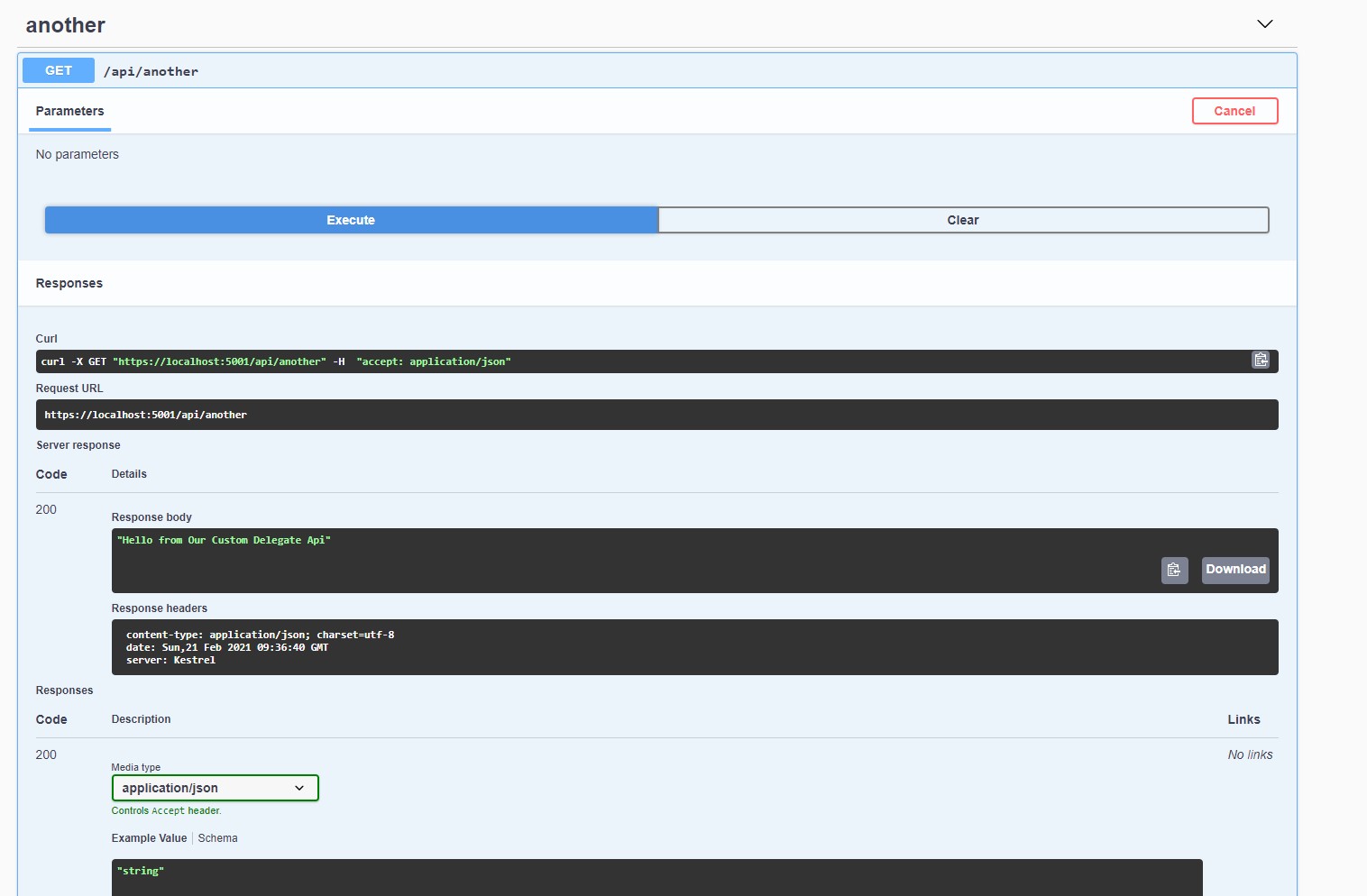
API Framework makes it easy to create OpenAPI based integration layers using ASP.NET Core
Add databases, OpenAPI & SOAP endpoints and more
API Framework extends ASP.NET Core with ability to easily create OpenAPI based integration layers. You can expose SQL Server databases, other OpenAPI Endpoints, local files and many more system through a single OpenAPI endpoint.
Reusability and plugins are at the core of API Framework and there’s currently more that 10 open source APIs available from Github & Nuget.org. These allow you to add many different endpoints into your system.
Configure using appsettings.json or through code
Endpoints can be added using the standard IConfiguration mechanism in ASP.NET Core. This means apps can use appsettings.json or any other IConfiguration source.
In addition to configuration files, endpoints and APIs can be configured using code. Or even conventions: With auto mode API Framework can auto resolve both the APIs and the endpoints based on configurable conventions.